How Builders Can Make improvements to Their Debugging Techniques By Gustavo Woltmann
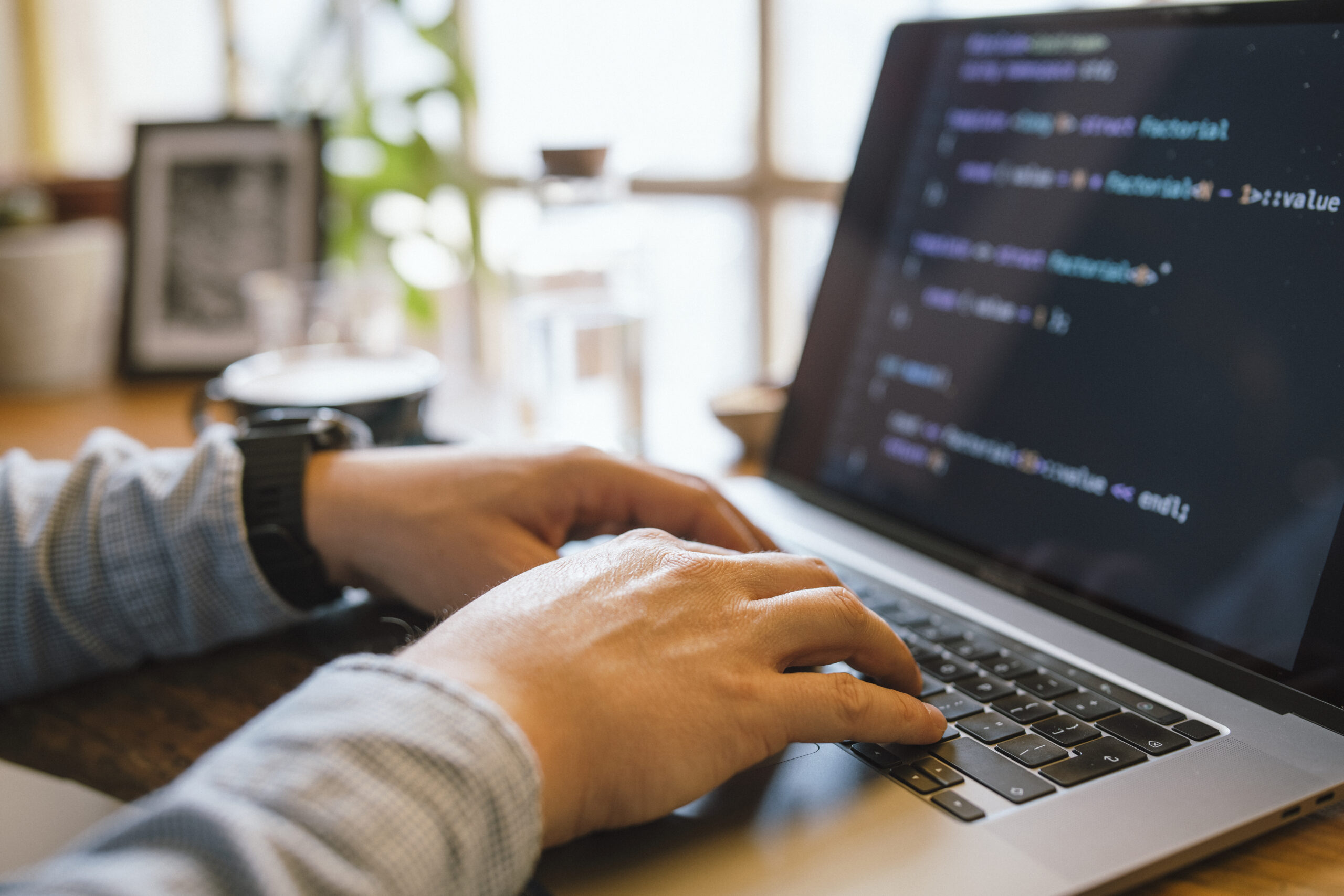
Debugging is One of the more important — nevertheless normally overlooked — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go Completely wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Listed here are several strategies to help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Resources
One of the fastest strategies developers can elevate their debugging expertise is by mastering the tools they use every day. Though producing code is one particular Portion of improvement, knowing ways to communicate with it successfully during execution is Similarly crucial. Modern enhancement environments appear equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these applications can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, step by code line by line, and in some cases modify code around the fly. When made use of effectively, they Allow you to notice just how your code behaves throughout execution, which is a must have for monitoring down elusive bugs.
Browser developer equipment, like Chrome DevTools, are indispensable for entrance-stop builders. They enable you to inspect the DOM, monitor community requests, view true-time performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can change disheartening UI problems into workable tasks.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control in excess of functioning processes and memory management. Understanding these instruments may have a steeper Finding out curve but pays off when debugging general performance issues, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into snug with version Handle devices like Git to understand code background, locate the precise minute bugs ended up released, and isolate problematic changes.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when issues crop up, you’re not shed in the dark. The greater you know your applications, the greater time you could expend resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and often overlooked — steps in helpful debugging is reproducing the condition. In advance of jumping in to the code or making guesses, builders will need to make a steady atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of likelihood, often bringing about wasted time and fragile code changes.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it gets to be to isolate the precise situations less than which the bug happens.
As you’ve collected more than enough data, try to recreate the situation in your local setting. This could suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, take into consideration composing automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only support expose the condition but additionally protect against regressions in the future.
At times, The problem may be surroundings-precise — it'd occur only on specified functioning systems, browsers, or below distinct configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can continuously recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You should use your debugging resources a lot more properly, examination likely fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract complaint into a concrete challenge — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. In lieu of viewing them as aggravating interruptions, developers should really master to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the concept very carefully As well as in whole. Several builders, particularly when below time tension, glance at the first line and straight away start off creating assumptions. But further inside the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them very first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and point you toward the liable code.
It’s also useful to be familiar with the terminology in the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Mastering to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context through which the mistake happened. Verify connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it provides true-time insights into how an application behaves, aiding you recognize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with knowing what to log and at what amount. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for standard gatherings (like profitable start off-ups), WARN for potential challenges that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the technique can’t proceed.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, state variations, enter/output values, and critical final decision factors in your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it is a sort of investigation. To effectively determine and correct bugs, builders ought to solution the process like a detective fixing a thriller. This frame of mind can help stop working complex problems into manageable components and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent details as it is possible to with no jumping to conclusions. Use logs, check circumstances, and user reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Question oneself: What could possibly be creating this behavior? Have any variations a short while ago been designed on the codebase? Has this concern occurred before less than very similar conditions? The objective would be to slender down options and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed setting. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out near awareness to tiny particulars. Bugs often cover in the minimum expected destinations—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be comprehensive and client, resisting the urge to patch the issue devoid of completely being familiar with it. Short term fixes may perhaps conceal the actual issue, only for it to resurface afterwards.
Finally, retain notes on what you tried out and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for foreseeable future issues and aid Some others understand your reasoning.
By pondering similar to a detective, developers can sharpen their analytical techniques, approach issues methodically, and turn into more effective at uncovering hidden difficulties in complex techniques.
Publish Checks
Writing tests is one of the best strategies to transform your debugging skills and General advancement effectiveness. Exams not simply enable capture bugs early but will also function a security Web that offers you confidence when making modifications in your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint specifically the place and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These tiny, isolated exams can swiftly reveal whether or not a particular piece of logic is working as envisioned. Every time a take a look at fails, you promptly know the place to seem, drastically minimizing time invested debugging. Device assessments are Specially beneficial for catching regression bugs—concerns that reappear following Beforehand staying mounted.
Up coming, integrate integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous aspects of your software function together efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If a thing breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Composing tests also forces you to definitely think critically about your code. To check a attribute properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the test fails continuously, you'll be able to center on fixing the bug and observe your take a look at pass when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing game into a structured and predictable approach—serving to you capture more bugs, more quickly and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for also extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours before. During this point out, your Mind gets significantly less effective at issue-solving. A brief stroll, a coffee break, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly see a lacking semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, stretch, or do something unrelated to code. It might experience counterintuitive, Specially less than restricted deadlines, but it really truly causes quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you stay away from the tunnel eyesight that often blocks your progress. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can instruct you a little something beneficial should you make time to replicate and review what went wrong.
Begin by asking your self several essential issues when the bug is solved: What prompted it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make more robust coding behaviors transferring forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, Gustavo Woltmann AI you’ll get started appreciating them as crucial parts of your growth journey. In the end, a lot of the greatest builders usually are not those who generate excellent code, but those who continually master from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.